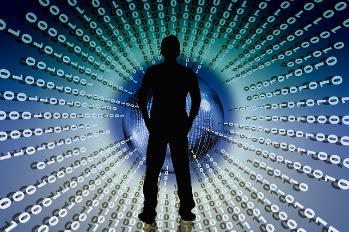
Tkinterで使われるscrollbarとは?活用事例を交えて徹底解説
今回はTkinterで使われるscrollbarに関して、活用事例を交えて徹底解説いたします。Tkinterのscrollbarがわからない、実例を通して学びたい方へおすすめです。是非最後まで一読ください。
- そもそもTkinterで使われるscrollbarとは?
- Tkinterの構成要素
- scrollbar Widgetを活用できる条件
- scrollbar Widgetの設定手順
- Tkinterで活用されるscrollbar Widgetの定義
- その他のWidgetを使った、scrollbar Widgetサンプル集
- まとめ
- 参考文献
執筆者 - おすすめの記事3選
そもそもTkinterで使われるscrollbarとは?
Tkinterで使われるscrollbarとは、Widgetの一種で、パソコン操作画面の右側または下側に表示される棒状の操作ツールを意味します。
具体的に画像を通して説明すると、
ピンク色で囲まれる箇所をscrollbarと呼びます。
また右側にあるscrollbarを垂直scrollbar、下側にあるscrollbarを水平scrollbar、scrollbarそのものをscrollbar Widgetと呼んだりします。
scrollbarを左右または上下へ動かすことで、既定表示サイズを超えた内容を閲覧できます。
聞き慣れない言葉がいくつか出てきましたね。。以下の「Tkinterの構成要素」を確認しながら、情報を整理しましょう。
Tkinterの構成要素
Tkinterの構成要素として、Window, Frame, Widgetの概念が存在します。
名称 | 説明文 |
---|---|
Window | 画像のオレンジ色枠の部分になります。Tkinter画面全体を表します。 |
Frame | 画像内の青色枠部分になります。Widgetが1つ以上ある場合に、取りまとめるものです。 |
Widget | 緑色枠, ピンク枠で囲まれる部分になります。1つの機能を持つ最小単位 = Widgetと考えると良いでしょう。 |
前の章でTkinterで活用されるscrollbarとは、Widgetの一種で、パソコン操作画面の右側または下側に表示される棒状の操作ツールとお伝えしました。
「Tkinterの構成要素」の画像内では、緑色枠に該当するWidgetに対して、編集することになります。
Tkinterの構成要素を理解したところで、実際にscrollbar Widgetの活用方法を理解していきましょう。
scrollbar Widgetを活用できる条件
scrollbar Widgetはその他のWidgetと異なり、単体では活用できません。
つまり、その他のWidgetに紐付けて利用することになります。
紐づけて利用できるWidgetの種類としては、
の7種類があります。
各種Widgetに関する詳細情報は、それぞれ以下の記事でまとめておりますので、是非ご確認ください。
scrollbar Widgetの設定手順
先ほどはscrollbar Widgetは単体では利用できず、その他のWidgetに紐づけて利用することをお伝えしました。
「scrollbar Widgetの設定手順」では、scrollbar Widgetをどのように設定するのか、コードを踏まえて解説いたします。
「scrollbar Widgetの設定手順」を説明するため、紐づけるWidget先としてtext Widgetを活用します。
scrollbar Widgetの設定手順につきましては、
- text Widgetの作成
- scrollbar Widgetの作成
- scrollbar Widgetをtext Widgetに反映する
- text Widget, scrollbar Widgetを配置
で行います。順に見ていきましょう。
text Widgetの作成
scrollbar Widgetの紐づけ先となる、text Widgetを作成します。
1import tkinter as tk
2
3class Application(tk.Frame):
4 def __init__(self, master=None):
5 # Windowの初期設定を行う。
6 super().__init__(master)
7
8 # Windowの画面サイズを設定する。
9 # geometryについて : https://kuroro.blog/python/rozH3S2CYE0a0nB3s2QL/
10 self.master.geometry("300x200")
11
12 # Windowを親要素として、frame Widget(Frame)を作成する。
13 # Frameについて : https://kuroro.blog/python/P20XOidA5nh583fYRvxf/
14 frame = tk.Frame(self.master)
15
16 # Windowを親要素とした場合に、frame Widget(Frame)をどのように配置するのか?
17 # packについて : https://kuroro.blog/python/UuvLfIBIEaw98BzBZ3FJ/
18 frame.pack()
19
20 # 1. text Widgetの作成
21 # frame Widget(Frame)を親要素として、text Widgetを作成する。
22 # height : 高さを設定
23 # Textについて : https://kuroro.blog/python/bK6fWsP9LMqmER1CBz9E/
24 text = tk.Text(frame, height=4)
25
26# Tkinter初学者参考 : https://docs.python.org/ja/3/library/tkinter.html#a-simple-hello-world-program
27if __name__ == "__main__":
28 # Windowを生成する。
29 # Windowについて : https://kuroro.blog/python/116yLvTkzH2AUJj8FHLx/
30 root = tk.Tk()
31 app = Application(master=root)
32 # Windowをループさせて、継続的にWindow表示させる。
33 # mainloopについて : https://kuroro.blog/python/DmJdUb50oAhmBteRa4fi/
34 app.mainloop()
ポイントは、text Widgetに対してheight optionを設定している点です。高さの指定がないと、scrollbar Widgetを動かすことができず、不十分な結果となってしまいます。
scrollbar Widgetの作成
scrollbar Widgetの作成には、tk.Scrollbar()
を使用します。「text Widgetの作成」で紹介したコード内のtext = tk.Text(frame, height=4)
の下へ以下のコードを貼り付けてください。
1# 2. scrollbar Widgetの作成
2# frame Widget(Frame)を親要素として、scrollbar Widgetを作成する。
3# 第一引数 : 親Widget
4# 第二引数以降(任意) : option
5# orient option : 垂直scrollbarを作成するため、tk.VERTICALを設定。水平scrollbarの場合は、tk.HORIZONTALを設定する。
6# command option : scrollbar Widgetを動かした場合に、連動して表示する内容を設定。今回は、text Widgetをy軸方向へ動かした内容を表示する。
7scrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview)
tk.Scrollbar()
の第一引数に親Widget、第二引数以降にoptionを指定します。
今回は垂直scrollbarを実現するために、orient optionにtk.VERTICALを設定。
またcommand optionへ垂直scrollbarを動かした場合に、連動して表示する内容をtext.yviewと設定しました。(水平scrollbarの場合、text.xviewを設定する)
scrollbar Widgetをtext Widgetに反映する
scrollbar Widgetをtext Widgetに反映します。「scrollbar Widgetの作成」で紹介したコード内のscrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview)
の下へ以下のコードを貼り付けてください。
1# 3. scrollbar Widgetをtext Widgetに反映する
2# scrollbar Widgetの設定内容をtext Widgetと紐付ける。
3# yscrollcommand : text Widget内で上下移動した場合に、scrollbarが追従するように設定する。
4text["yscrollcommand"] = scrollbar.set
text Widgetのyscrollcommand optionへscrollbar.set
を設定します。
これによりtext Widgetとscrollbar Widgetが紐づき、scrollbar Widgetを用いて、内容を確認できるようになります。
text Widget, scrollbar Widgetを配置
最後にtext Widget, scrollbar Widgetの配置を行います。「scrollbar Widgetをtext Widgetに反映する」で紹介したコード内のtext["yscrollcommand"] = scrollbar.set
の下へ以下のコードを貼り付けてください。
1# 4. text Widget, scrollbar Widgetを配置
2# frame Widget(Frame)を親要素とした場合に、text Widgetをどのように配置するのか?
3# gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
4text.grid(row=0, column=0)
5# frame Widget(Frame)を親要素とした場合に、scrollbar Widgetをどのように配置するのか?
6# gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
7scrollbar.grid(row=0, column=1, sticky=(tk.N, tk.S))
以上をもって、scrollbarを利用できるようになりました。
実際に紹介したコードを、組み合わせて実行すると、以下の動画のような結果が得られます。
完成コード
1import tkinter as tk
2
3class Application(tk.Frame):
4 def __init__(self, master=None):
5 # Windowの初期設定を行う。
6 super().__init__(master)
7
8 # Windowの画面サイズを設定する。
9 # geometryについて : https://kuroro.blog/python/rozH3S2CYE0a0nB3s2QL/
10 self.master.geometry("300x200")
11
12 # Windowを親要素として、frame Widget(Frame)を作成する。
13 # Frameについて : https://kuroro.blog/python/P20XOidA5nh583fYRvxf/
14 frame = tk.Frame(self.master)
15
16 # Windowを親要素とした場合に、frame Widget(Frame)をどのように配置するのか?
17 # packについて : https://kuroro.blog/python/UuvLfIBIEaw98BzBZ3FJ/
18 frame.pack()
19
20 # 1. text Widgetの作成
21 # frame Widget(Frame)を親要素として、text Widgetを作成する。
22 # height : 高さを設定
23 # Textについて : https://kuroro.blog/python/bK6fWsP9LMqmER1CBz9E/
24 text = tk.Text(frame, height=4)
25
26 # 2. scrollbar Widgetの作成
27 # frame Widget(Frame)を親要素として、scrollbar Widgetを作成する。
28 # orient option : 垂直scrollbarを作成するため、tk.VERTICALを設定。水平scrollbarの場合は、tk.HORIZONTALを設定する。
29 # command option : scrollbar Widgetを動かした場合に、連動して表示する内容を設定。今回は、text Widgetをy軸方向へ動かした内容を表示する。
30 scrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview)
31
32 # 3. scrollbar Widgetをtext Widgetに反映する
33 # scrollbar Widgetの設定内容をtext Widgetと紐付ける。
34 # yscrollcommand : text Widget内で上下移動した場合に、scrollbarが追従するように設定する。
35 text["yscrollcommand"] = scrollbar.set
36
37 # 4. text Widget, scrollbar Widgetを配置
38 # frame Widget(Frame)を親要素とした場合に、text Widgetをどのように配置するのか?
39 # gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
40 text.grid(row=0, column=0)
41 # frame Widget(Frame)を親要素とした場合に、scrollbar Widgetをどのように配置するのか?
42 # gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
43 scrollbar.grid(row=0, column=1, sticky=(tk.N, tk.S))
44
45# Tkinter初学者参考 : https://docs.python.org/ja/3/library/tkinter.html#a-simple-hello-world-program
46if __name__ == "__main__":
47 # Windowを生成する。
48 # Windowについて : https://kuroro.blog/python/116yLvTkzH2AUJj8FHLx/
49 root = tk.Tk()
50 app = Application(master=root)
51 # Windowをループさせて、継続的にWindow表示させる。
52 # mainloopについて : https://kuroro.blog/python/DmJdUb50oAhmBteRa4fi/
53 app.mainloop()
Tkinterで活用されるscrollbar Widgetの定義
scrollbar Widgetは、
1tk.Scrollbar('親要素', option1, option2, ...)
で定義されます。
scrollbar Widgetで使われるoptionの種類としては
- command, orient
- borderwidth, bd
- background, bg
- width
- cursor
- activebackground
- relief
- activerelief
- highlightcolor, highlightbackground, highlightthickness
- repeatdelay repeatinterval
- jump
があります。順番に見ていきましょう。
※ optionの種類一覧を調べたい場合は、以下のようにコードを記述してご確認ください。
1import tkinter as tk
2
3# scrollbarを生成する。
4scrollbar = tk.Scrollbar()
5# scrollbarに関するoptionの種類一覧を取得する。
6print(scrollbar.keys())
command, orient
command option, orient optionに関しては、「scrollbar Widgetの作成」のコード内で解説しましたので、ご確認ください。
borderwidth, bd
borderwidth option, bd optionを利用すると、scrollbar Widgetの枠の大きさを設定します。
borderwidthとbd両方のoptionを用いて、値を設定した場合、後ろの引数に設定されるoptionが優先されます。
例えば「完成コード」で紹介したscrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview)
箇所を、以下のように変更すると、
1# 別解法 ##########################
2# scrollbar = tk.Scrollbar(frame)
3# scrollbar.config(orient=tk.VERTICAL, command=text.yview, bd=10) or scrollbar.configure(orient=tk.VERTICAL, command=text.yview, bd=10)
4##################################
5scrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview, bd=10)
以下の画像のようにscrollbar Widgetを描画します。
background, bg
background option, bg optionを利用すると、scrollbar Widgetの背景色を設定します。
backgroundとbg両方のoptionを用いて、値を設定した場合、後ろの引数に設定されるoptionが優先されます。
色に関しては、Tkinterの色の使い方とは?活用例から色の一覧をまとめて紹介!?で総括していますので、詳しく知りたい方は是非ご確認ください。
例えば「完成コード」で紹介したscrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview)
箇所を、以下のように変更すると、
1# 別解法 ##########################
2# scrollbar = tk.Scrollbar(frame)
3# scrollbar.config(orient=tk.VERTICAL, command=text.yview, bd=20, background='red') or scrollbar.configure(orient=tk.VERTICAL, command=text.yview, bd=20, background='red')
4##################################
5# 色について : https://kuroro.blog/python/YcZ6Yh4PswqUzaQXwnG2/
6scrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview, bd=20, background='red')
以下の画像のようにscrollbar Widgetを描画します。
width
width optionを利用すると、scrollbar Widgetの大きさを設定できます。
例えば「完成コード」で紹介したscrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview)
箇所を、以下のように変更すると、
1# 別解法 ##########################
2# scrollbar = tk.Scrollbar(frame)
3# scrollbar.config(orient=tk.VERTICAL, command=text.yview, width=30) or scrollbar.configure(orient=tk.VERTICAL, command=text.yview, width=30)
4##################################
5scrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview, width=30)
以下の画像のようにscrollbar Widgetを描画します。
cursor
cursor optionを利用すると、scrollbar Widget内へマウスカーソルを移動すると矢印の見た目を変化できます。
見た目のバリエーションについてはこちらのサイトにまとまっていますので、ご確認ください。
例えば「完成コード」で紹介したscrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview)
箇所を、以下のように変更して、
1# 別解法 ##########################
2# scrollbar = tk.Scrollbar(frame)
3# scrollbar.config(orient=tk.VERTICAL, command=text.yview, cursor="clock") or scrollbar.configure(orient=tk.VERTICAL, command=text.yview, cursor="clock")
4##################################
5scrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview, cursor="clock")
scrollbar Widget内へマウスカーソルを移動すると、矢印の見た目が変更します。
activebackground
activebackground optionを設定すると、scrollbar Widgetへマウスカーソルが移動した場合の背景色を変更できます。
※ 2021年7月4日現在、筆者のMac OSではactivebackground optionを設定しても、scrollbar Widgetは変更されませんでした。
relief
relief optionを利用すると、scrollbar Widgetの枠のデザインを設定できます。
指定方法としては、
- tk.RAISED
- tk.SUNKEN
- tk.FLAT
- tk.RIDGE
- tk.GROOVE
- tk.SOLID
の6種類があります。
例えば「完成コード」で紹介したコードを、以下のように変更すると、
1def __init__(self, master=None):
2 ...
3 frame.pack()
4
5- text = tk.Text(frame, height=4)
6
7+ reliefList = [tk.RAISED, tk.SUNKEN, tk.FLAT, tk.RIDGE, tk.GROOVE, tk.SOLID]
8+ i = 0
9+ for relief in reliefList:
10+ text = tk.Text(frame, height=4)
11
12- scrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview)
13+ scrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview, relief=relief, bd=10)
14
15- text["yscrollcommand"] = scrollbar.set
16+ text["yscrollcommand"] = scrollbar.set
17
18- text.grid(row=0, column=0)
19+ text.grid(row=i, column=0)
20
21- scrollbar.grid(row=0, column=1, sticky=(tk.N, tk.S))
22+ scrollbar.grid(row=i, column=1, sticky=(tk.N, tk.S))
23
24+ i = i + 1
以下の画像のようにscrollbar Widgetを描画します。
activerelief
activerelief optionを利用すると、scrollbar Widgetへマウスカーソルが移動した場合の、scrollbar Widgetの枠のデザインを設定できます。
※ 2021年7月4日現在、筆者のMac OSではactiverelief optionを設定しても、scrollbar Widgetは変更されませんでした。
highlightcolor, highlightbackground, highlightthickness
highlightcolor option, highlightbackground option, highlightthickness optionを利用すると、それぞれフォーカスがあてられた時の囲い線の色, フォーカスが外れた時の囲い線の色, 囲い線の太さを設定します。
例えば「完成コード」で紹介したscrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview)
箇所を、以下のように変更すると、
1# 別解法 ##########################
2# scrollbar = tk.Scrollbar(frame)
3# scrollbar.config(orient=tk.VERTICAL, command=text.yview, highlightbackground="red", highlightthickness=20) or scrollbar.configure(orient=tk.VERTICAL, command=text.yview, highlightbackground="red", highlightthickness=20)
4##################################
5# 色について : https://kuroro.blog/python/YcZ6Yh4PswqUzaQXwnG2/
6scrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview, highlightbackground="red", highlightthickness=20)
以下の画像のようにscrollbar Widgetを描画します。
また「完成コード」で紹介したscrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview)
箇所を、以下のように変更すると、
1# 色について : https://kuroro.blog/python/YcZ6Yh4PswqUzaQXwnG2/
2scrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview, , highlightcolor='blue', highlightthickness=20)
3# scrollbar Widgetへfocusを与える。
4scrollbar.focus()
以下の画像のようにscrollbar Widgetを描画します。
repeatdelay, repeatinterval
repeatdelay option, repeatinterval optionを利用すると、scrollbarを長押ししている最中に、関数(command option)を呼び出すことができます。
repeatdelay optionでは1回目の関数(command option)呼び出しの時間を遅らせること(ミリ秒で設定)ができます。
repeatinterval optionでは何秒間隔(ミリ秒で設定)で関数(command option)呼び出しするか設定できます。
例えば「完成コード」で紹介したscrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview)
箇所を、以下のように変更すると、
1# 別解法 ##########################
2# scrollbar = tk.Scrollbar(frame)
3# scrollbar.config(orient=tk.VERTICAL, command=text.yview, repeatdelay=800, repeatinterval=1000) or scrollbar.configure(orient=tk.VERTICAL, command=text.yview, repeatdelay=800, repeatinterval=1000)
4##################################
5scrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=text.yview, repeatdelay=800, repeatinterval=1000)
ボタン押下後800ミリ秒後に1回目の関数(command option)呼び出しを行い、その後1000ミリ秒ごとに関数(command option)呼び出しを行います。
jump
jump opitonを利用すると、scrollbar Widgetのつまみを用いて移動するときの、内容を表示するタイミングを変更できます。
具体的につまみを画像を通して説明すると、
ピンク色で囲まれる箇所をつまみと呼びます。
Trueの場合、つまみを離したタイミングで表示内容を変更する、False(デフォルト)の場合、つまみの移動と同期して表示内容を変更します。
その他のWidgetを使った、scrollbar Widgetサンプル集
最後にscrollbar Widgetサンプル集をご紹介いたします。それぞれコードと実行結果の画像をまとめておきますので、ご利用ください。
spinbox Widgetの場合
1import tkinter as tk
2
3class Application(tk.Frame):
4 def __init__(self, master=None):
5 # Windowの初期設定を行う。
6 super().__init__(master)
7
8 # Windowの画面サイズを設定する。
9 # geometryについて : https://kuroro.blog/python/rozH3S2CYE0a0nB3s2QL/
10 self.master.geometry("300x200")
11
12 # Windowを親要素として、frame Widget(Frame)を作成する。
13 # Frameについて : https://kuroro.blog/python/P20XOidA5nh583fYRvxf/
14 frame = tk.Frame(self.master)
15
16 # Windowを親要素とした場合に、frame Widget(Frame)をどのように配置するのか?
17 # packについて : https://kuroro.blog/python/UuvLfIBIEaw98BzBZ3FJ/
18 frame.pack()
19
20 # frame Widget(Frame)を親要素として、spinbox Widgetを作成する。
21 # width : 幅を設定
22 # spinboxについて : https://kuroro.blog/python/CQZWZZXhhyD3B1TWP3FN/
23 spinbox = tk.Spinbox(frame, width=50)
24
25 # frame Widget(Frame)を親要素として、scrollbar Widgetを作成する。
26 # orient option : 水平scrollbarを作成するため、tk.HORIZONTALを設定。
27 # command option : scrollbar Widgetを動かした場合に、連動して表示する内容を設定。今回は、spinbox Widgetをx軸方向へ動かした内容を表示する。
28 scrollbar = tk.Scrollbar(frame, orient=tk.HORIZONTAL, command=spinbox.xview)
29
30 # scrollbar Widgetの設定内容をspinbox Widgetと紐付ける。
31 # xscrollcommand : spinbox Widget内で左右移動した場合に、scrollbarが追従するように設定する。
32 spinbox["xscrollcommand"] = scrollbar.set
33
34 # frame Widget(Frame)を親要素とした場合に、spinbox Widgetをどのように配置するのか?
35 # gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
36 spinbox.grid(row=0, column=0)
37 # frame Widget(Frame)を親要素とした場合に、scrollbar Widgetをどのように配置するのか?
38 # gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
39 scrollbar.grid(row=1, column=0, sticky=(tk.W, tk.E))
40
41# Tkinter初学者参考 : https://docs.python.org/ja/3/library/tkinter.html#a-simple-hello-world-program
42if __name__ == "__main__":
43 # Windowを生成する。
44 # Windowについて : https://kuroro.blog/python/116yLvTkzH2AUJj8FHLx/
45 root = tk.Tk()
46 app = Application(master=root)
47 # Windowをループさせて、継続的にWindow表示させる。
48 # mainloopについて : https://kuroro.blog/python/DmJdUb50oAhmBteRa4fi/
49 app.mainloop()
listbox Widgetの場合
1import tkinter as tk
2
3class Application(tk.Frame):
4 def __init__(self, master=None):
5 # Windowの初期設定を行う。
6 super().__init__(master)
7
8 # Windowの画面サイズを設定する。
9 # geometryについて : https://kuroro.blog/python/rozH3S2CYE0a0nB3s2QL/
10 self.master.geometry("300x200")
11
12 # Windowを親要素として、frame Widget(Frame)を作成する。
13 # Frameについて : https://kuroro.blog/python/P20XOidA5nh583fYRvxf/
14 frame = tk.Frame(self.master)
15
16 # Windowを親要素とした場合に、frame Widget(Frame)をどのように配置するのか?
17 # packについて : https://kuroro.blog/python/UuvLfIBIEaw98BzBZ3FJ/
18 frame.pack()
19
20 # frame Widget(Frame)を親要素として、listbox Widgetを作成する。
21 # height : 高さを設定
22 # listvariable : 選択要素の初期化を行う。
23 # StringVarについて : https://kuroro.blog/python/K53voPjJuKFfYrjmP8FP/
24 # listboxについて : https://kuroro.blog/python/XMWVRR2MEZAe4bpPDDXE/
25 listbox = tk.Listbox(frame, height=5, listvariable=tk.StringVar(value=(1, 2, 3, 4, 5, 6, 7)))
26
27 # frame Widget(Frame)を親要素として、scrollbar Widgetを作成する。
28 # orient option : 垂直scrollbarを作成するため、tk.VERTICALを設定。
29 # command option : scrollbar Widgetを動かした場合に、連動して表示する内容を設定。今回は、listbox Widgetをy軸方向へ動かした内容を表示する。
30 scrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=listbox.yview)
31
32 # scrollbar Widgetの設定内容をlistbox Widgetと紐付ける。
33 # yscrollcommand : listbox Widget内で上下移動した場合に、scrollbarが追従するように設定する。
34 listbox["yscrollcommand"] = scrollbar.set
35
36 # frame Widget(Frame)を親要素とした場合に、listbox Widgetをどのように配置するのか?
37 # gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
38 listbox.grid(row=0, column=0)
39 # frame Widget(Frame)を親要素とした場合に、scrollbar Widgetをどのように配置するのか?
40 # gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
41 scrollbar.grid(row=0, column=1, sticky=(tk.N, tk.S))
42
43# Tkinter初学者参考 : https://docs.python.org/ja/3/library/tkinter.html#a-simple-hello-world-program
44if __name__ == "__main__":
45 # Windowを生成する。
46 # Windowについて : https://kuroro.blog/python/116yLvTkzH2AUJj8FHLx/
47 root = tk.Tk()
48 app = Application(master=root)
49 # Windowをループさせて、継続的にWindow表示させる。
50 # mainloopについて : https://kuroro.blog/python/DmJdUb50oAhmBteRa4fi/
51 app.mainloop()
canvas Widgetの場合
1import tkinter as tk
2
3class Application(tk.Frame):
4 def __init__(self, master=None):
5 # Windowの初期設定を行う。
6 super().__init__(master)
7
8 # Windowの画面サイズを設定する。
9 # geometryについて : https://kuroro.blog/python/rozH3S2CYE0a0nB3s2QL/
10 self.master.geometry("300x200")
11
12 # Windowを親要素として、frame Widget(Frame)を作成する。
13 # Frameについて : https://kuroro.blog/python/P20XOidA5nh583fYRvxf/
14 frame = tk.Frame(self.master)
15
16 # Windowを親要素とした場合に、frame Widget(Frame)をどのように配置するのか?
17 # packについて : https://kuroro.blog/python/UuvLfIBIEaw98BzBZ3FJ/
18 frame.pack()
19
20 # frame Widget(Frame)を親要素として、canvas Widgetを作成する。
21 # background : 背景色の設定
22 # 色について : https://kuroro.blog/python/YcZ6Yh4PswqUzaQXwnG2/
23 # width : 幅を設定
24 # height : 高さを設定
25 # scrollregion(w, n, e, s) : w : West, n : North, e : East, s : Southの略。
26 # 設定したwidth(幅)、height(高さ)以上にscrollできる領域を設けたい場合に、方位に合わせて値を設定する。
27 # 今回は、垂直方向へscrollさせたいので、sのみに値を設定する。
28 # yscrollincrement : scrollすることで新しく確認できるcanvas Widgetの領域を、1pxから100pxへ変更する設定
29 # canvasについて : https://kuroro.blog/python/V63iINoXI8iwMeRMEJPK/
30 canvas = tk.Canvas(frame, background="white", width=400, height=300, scrollregion=(0, 0, 0, 500), yscrollincrement=100)
31 # x1座標 : 200, y1座標 : 200, x2座標 : 10, y2座標 : 20, x3座標 : 60, y3座標 : 100, x4座標 : 1000, y4座標 : 1000
32 # option : fill(線を引くときの色を設定)
33 # 戻り値 : ID
34 # canvasについて : https://kuroro.blog/python/ANyM9WLpd0LSXRQAELOj/
35 canvas.create_line(200, 200, 10, 20, 60, 100, 1000, 1000, fill='black')
36
37 # frame Widget(Frame)を親要素として、scrollbar Widgetを作成する。
38 # orient option : 垂直scrollbarを作成するため、tk.VERTICALを設定。
39 # command option : scrollbar Widgetを動かした場合に、連動して表示する内容を設定。今回は、canvas Widgetをy軸方向へ動かした内容を表示する。
40 scrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=canvas.yview)
41
42 # scrollbar Widgetの設定内容をcanvas Widgetと紐付ける。
43 # yscrollcommand : canvas Widget内で上下移動した場合に、scrollbarが追従するように設定する。
44 canvas["yscrollcommand"] = scrollbar.set
45
46 # frame Widget(Frame)を親要素とした場合に、canvas Widgetをどのように配置するのか?
47 # gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
48 canvas.grid(row=0, column=0)
49 # frame Widget(Frame)を親要素とした場合に、scrollbar Widgetをどのように配置するのか?
50 # gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
51 scrollbar.grid(row=0, column=1, sticky=(tk.N, tk.S))
52
53# Tkinter初学者参考 : https://docs.python.org/ja/3/library/tkinter.html#a-simple-hello-world-program
54if __name__ == "__main__":
55 # Windowを生成する。
56 # Windowについて : https://kuroro.blog/python/116yLvTkzH2AUJj8FHLx/
57 root = tk.Tk()
58 app = Application(master=root)
59 # Windowをループさせて、継続的にWindow表示させる。
60 # mainloopについて : https://kuroro.blog/python/DmJdUb50oAhmBteRa4fi/
61 app.mainloop()
entry Widgetの場合
1import tkinter as tk
2
3class Application(tk.Frame):
4 def __init__(self, master=None):
5 # Windowの初期設定を行う。
6 super().__init__(master)
7
8 # Windowの画面サイズを設定する。
9 # geometryについて : https://kuroro.blog/python/rozH3S2CYE0a0nB3s2QL/
10 self.master.geometry("300x200")
11
12 # Windowを親要素として、frame Widget(Frame)を作成する。
13 # Frameについて : https://kuroro.blog/python/P20XOidA5nh583fYRvxf/
14 frame = tk.Frame(self.master)
15
16 # Windowを親要素とした場合に、frame Widget(Frame)をどのように配置するのか?
17 # packについて : https://kuroro.blog/python/UuvLfIBIEaw98BzBZ3FJ/
18 frame.pack()
19
20 # frame Widget(Frame)を親要素として、entry Widgetを作成する。
21 # width : 幅を設定
22 # Entryについて : https://kuroro.blog/python/PUZp77YFxrXvMCjpZbUg/
23 entry = tk.Entry(frame, width=50)
24
25 # frame Widget(Frame)を親要素として、scrollbar Widgetを作成する。
26 # orient option : 水平scrollbarを作成するため、tk.HORIZONTALを設定。
27 # command option : scrollbar Widgetを動かした場合に、連動して表示する内容を設定。今回は、entry Widgetをx軸方向へ動かした内容を表示する。
28 scrollbar = tk.Scrollbar(frame, orient=tk.HORIZONTAL, command=entry.xview)
29
30 # scrollbar Widgetの設定内容をentry Widgetと紐付ける。
31 # xscrollcommand : entry Widget内で左右移動した場合に、scrollbarが追従するように設定する。
32 entry["xscrollcommand"] = scrollbar.set
33
34 # frame Widget(Frame)を親要素とした場合に、entry Widgetをどのように配置するのか?
35 # gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
36 entry.grid(row=0, column=0)
37 # frame Widget(Frame)を親要素とした場合に、scrollbar Widgetをどのように配置するのか?
38 # gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
39 scrollbar.grid(row=1, column=0, sticky=(tk.W, tk.E))
40
41# Tkinter初学者参考 : https://docs.python.org/ja/3/library/tkinter.html#a-simple-hello-world-program
42if __name__ == "__main__":
43 # Windowを生成する。
44 # Windowについて : https://kuroro.blog/python/116yLvTkzH2AUJj8FHLx/
45 root = tk.Tk()
46 app = Application(master=root)
47 # Windowをループさせて、継続的にWindow表示させる。
48 # mainloopについて : https://kuroro.blog/python/DmJdUb50oAhmBteRa4fi/
49 app.mainloop()
combobox Widgetの場合
1import tkinter as tk
2from tkinter import *
3from tkinter import ttk
4
5class Application(tk.Frame):
6 def __init__(self, master=None):
7 # Windowの初期設定を行う。
8 super().__init__(master)
9
10 # Windowの画面サイズを設定する。
11 # geometryについて : https://kuroro.blog/python/rozH3S2CYE0a0nB3s2QL/
12 self.master.geometry("300x200")
13
14 # Windowを親要素として、frame Widget(Frame)を作成する。
15 # Frameについて : https://kuroro.blog/python/P20XOidA5nh583fYRvxf/
16 frame = tk.Frame(self.master)
17
18 # Windowを親要素とした場合に、frame Widget(Frame)をどのように配置するのか?
19 # packについて : https://kuroro.blog/python/UuvLfIBIEaw98BzBZ3FJ/
20 frame.pack()
21
22 # frame Widget(Frame)を親要素として、combobox Widgetを作成する。
23 # width : 幅を設定
24 # values : 表示される選択肢の内容を設定
25 # Comboboxについて : https://kuroro.blog/python/3ZzPkezBOeTN7lletMyG/
26 combobox = ttk.Combobox(frame, width=10, values=('バイキンマン', '食パンまん'))
27
28 # frame Widget(Frame)を親要素として、scrollbar Widgetを作成する。
29 # orient option : 水平scrollbarを作成するため、tk.HORIZONTALを設定。
30 # command option : scrollbar Widgetを動かした場合に、連動して表示する内容を設定。今回は、combobox Widgetをx軸方向へ動かした内容を表示する。
31 scrollbar = tk.Scrollbar(frame, orient=tk.HORIZONTAL, command=combobox.xview)
32
33 # scrollbar Widgetの設定内容をcombobox Widgetと紐付ける。
34 # xscrollcommand : combobox Widget内で左右移動した場合に、scrollbarが追従するように設定する。
35 combobox["xscrollcommand"] = scrollbar.set
36
37 # frame Widget(Frame)を親要素とした場合に、combobox Widgetをどのように配置するのか?
38 # gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
39 combobox.grid(row=0, column=0)
40 # frame Widget(Frame)を親要素とした場合に、scrollbar Widgetをどのように配置するのか?
41 # gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
42 scrollbar.grid(row=1, column=0, sticky=(tk.W, tk.E))
43
44# Tkinter初学者参考 : https://docs.python.org/ja/3/library/tkinter.html#a-simple-hello-world-program
45if __name__ == "__main__":
46 # Windowを生成する。
47 # Windowについて : https://kuroro.blog/python/116yLvTkzH2AUJj8FHLx/
48 root = tk.Tk()
49 app = Application(master=root)
50 # Windowをループさせて、継続的にWindow表示させる。
51 # mainloopについて : https://kuroro.blog/python/DmJdUb50oAhmBteRa4fi/
52 app.mainloop()
treeview Widgetの場合
1import tkinter as tk
2from tkinter import ttk
3
4class Application(tk.Frame):
5 # treeview Widgetの情報を格納する変数
6 treeview = None
7
8 def __init__(self, master=None):
9 # Windowの初期設定を行う。
10 super().__init__(master)
11
12 # Windowの画面サイズを設定する。
13 # geometryについて : https://kuroro.blog/python/rozH3S2CYE0a0nB3s2QL/
14 self.master.geometry("300x200")
15
16 # Windowを親要素として、frame Widget(Frame)を作成する。
17 # Frameについて : https://kuroro.blog/python/P20XOidA5nh583fYRvxf/
18 frame = tk.Frame(self.master)
19
20 # Windowを親要素とした場合に、frame Widget(Frame)をどのように配置するのか?
21 # packについて : https://kuroro.blog/python/UuvLfIBIEaw98BzBZ3FJ/
22 frame.pack()
23
24 # frame Widget(Frame)を親要素として、treeview Widgetを作成する。
25 # columns : 各データ列へ名前を設定。
26 # Treeviewについて : https://kuroro.blog/python/ApqhLmDSo5OJwuetvTvy/
27 self.treeview = ttk.Treeview(frame, columns=["colA", "colB", "colC"])
28
29 #############################################
30 # <各列に対してオプション設定>
31 # #0 : 階層列(ツリーカラム)を意味する。
32 # 階層列(ツリーカラム)のオプションを設定。
33 # width : 幅の設定
34 self.treeview.column("#0", width=100)
35 # colA列のオプションを設定。
36 # width : 幅の設定
37 self.treeview.column("colA", width=80)
38 # colB列のオプションを設定。
39 # width : 幅の設定
40 self.treeview.column("colB", width=80)
41 # colC列のオプションを設定。
42 # width : 幅の設定
43 self.treeview.column("colC", width=80)
44 #############################################
45
46 #############################################
47 # <各列の見出しに対してオプション設定>
48 # #0 : 階層列(ツリーカラム)を意味する。
49 # 階層列(ツリーカラム)の見出しを設定。階層列とする。
50 self.treeview.heading("#0", text="階層列")
51 # colA列の見出しを設定。データ列Aとする。
52 self.treeview.heading("colA", text="データ列A")
53 # colB列の見出しを設定。データ列Bとする。
54 self.treeview.heading("colB", text="データ列B")
55 # colC列の見出しを設定。データ列Cとする。
56 self.treeview.heading("colC", text="データ列C")
57 #############################################
58
59 #############################################
60 # <アイテムを挿入>
61 # 第一引数 : 階層化する場合、親要素のアイテムIDを指定。階層化しない場合、""を指定する。
62 # 第二引数 : どのindex(アイテム位置)へアイテムを挿入するのか指定する。tk.END : index(アイテムの最終位置)
63 # text option : 階層列へ表示するアイテムの名前を設定。
64 # open option : 子要素のアイテムを展開して表示するのかどうか設定する。True : 子要素のアイテムを展開して表示する, False : 子要素のアイテムを展開して表示しない、デフォルト。
65 # values option : データ列へ表示する値を設定。
66 # 戻り値 : アイテムID
67 itemAId = self.treeview.insert("", tk.END, text="itemA", open=True, values=("data1a", "data1b", "data1c"))
68 # 第一引数 : 階層化する場合、親要素のアイテムIDを指定。階層化しない場合、""を指定する。
69 # 第二引数 : どのindex(アイテム位置)へアイテムを挿入するのか指定する。tk.END : index(アイテムの最終位置)
70 # text option : 階層列へ表示するアイテムの名前を設定。
71 # open option : 子要素のアイテムを展開して表示するのかどうか設定する。True : 子要素のアイテムを展開して表示する, False : 子要素のアイテムを展開して表示しない、デフォルト。
72 # values option : データ列へ表示する値を設定。
73 # 戻り値 : アイテムID
74 itemBId = self.treeview.insert(itemAId, tk.END, text="itemB", open=True, values=("data2a", "data2b", "data2c"))
75 # 第一引数 : 階層化する場合、親要素のアイテムIDを指定。階層化しない場合、""を指定する。
76 # 第二引数 : どのindex(アイテム位置)へアイテムを挿入するのか指定する。tk.END : index(アイテムの最終位置)
77 # text option : 階層列へ表示するアイテムの名前を設定。
78 # values option : データ列へ表示する値を設定。
79 # 戻り値 : アイテムID
80 itemCId = self.treeview.insert(itemBId, tk.END, text="itemC", values=("data3a", "data3b", "data3c"))
81 # 第一引数 : 階層化する場合、親要素のアイテムIDを指定。階層化しない場合、""を指定する。
82 # 第二引数 : どのindex(アイテム位置)へアイテムを挿入するのか指定する。tk.END : index(アイテムの最終位置)
83 # text option : 階層列へ表示するアイテムの名前を設定。
84 # values option : データ列へ表示する値を設定。
85 # 戻り値 : アイテムID
86 itemDId = self.treeview.insert("", tk.END, text="itemD", values=("data4a", "data4b", "data4c"))
87 itemEId = self.treeview.insert("", tk.END, text="itemE", values=("data5a", "data5b", "data5c"))
88 itemFId = self.treeview.insert("", tk.END, text="itemF", values=("data6a", "data6b", "data6c"))
89 itemGId = self.treeview.insert("", tk.END, text="itemG", values=("data7a", "data7b", "data7c"))
90 itemHId = self.treeview.insert("", tk.END, text="itemH", values=("data8a", "data8b", "data8c"))
91 itemIId = self.treeview.insert("", tk.END, text="itemI", values=("data9a", "data9b", "data9c"))
92 itemJId = self.treeview.insert("", tk.END, text="itemJ", values=("data10a", "data10b", "data10c"))
93 itemKId = self.treeview.insert("", tk.END, text="itemK", values=("data11a", "data11b", "data11c"))
94 itemLId = self.treeview.insert("", tk.END, text="itemL", values=("data12a", "data12b", "data12c"))
95 itemMId = self.treeview.insert("", tk.END, text="itemM", values=("data13a", "data13b", "data13c"))
96 itemNId = self.treeview.insert("", tk.END, text="itemN", values=("data14a", "data14b", "data14c"))
97 #############################################
98
99 # frame Widget(Frame)を親要素として、scrollbar Widgetを作成する。
100 # orient option : 垂直scrollbarを作成するため、tk.VERTICALを設定。
101 # command option : scrollbar Widgetを動かした場合に、連動して表示する内容を設定。今回は、treeview Widgetをy軸方向へ動かした内容を表示する。
102 scrollbar = tk.Scrollbar(frame, orient=tk.VERTICAL, command=self.treeview.yview)
103
104 # scrollbar Widgetの設定内容をtreeview Widgetと紐付ける。
105 # yscrollcommand : treeview Widget内で上下移動した場合に、scrollbarが追従するように設定する。
106 self.treeview["yscrollcommand"] = scrollbar.set
107
108 # frame Widget(Frame)を親要素とした場合に、treeview Widgetをどのように配置するのか?
109 # gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
110 self.treeview.grid(row=0, column=0)
111 # frame Widget(Frame)を親要素とした場合に、scrollbar Widgetをどのように配置するのか?
112 # gridについて : https://kuroro.blog/python/JoaowDiUdLAOj3cSBxiX/
113 scrollbar.grid(row=0, column=1, sticky=(tk.N, tk.S))
114
115# Tkinter初学者参考 : https://docs.python.org/ja/3/library/tkinter.html#a-simple-hello-world-program
116if __name__ == "__main__":
117 # Windowを生成する。
118 # Windowについて : https://kuroro.blog/python/116yLvTkzH2AUJj8FHLx/
119 root = tk.Tk()
120 app = Application(master=root)
121 # Windowをループさせて、継続的にWindow表示させる。
122 # mainloopについて : https://kuroro.blog/python/DmJdUb50oAhmBteRa4fi/
123 app.mainloop()
まとめ
- Tkinterの構成要素として、Window, Frame, Widgetの概念が存在する。
- Tkinterで使われるscrollbarとは、Widgetの一種で、パソコン操作画面の右側または下側に表示される棒状の操作ツールを意味します。
- 右側にあるscrollbarを垂直scrollbar、下側にあるscrollbarを水平scrollbar、scrollbarそのものをscrollbar Widgetと呼んだりします。